Rust in the Linux kernel
There is now a serious attempt to bring a second language to the Linux kernel and earlier this month Miguel Ojeda put up an RFC for adding Rust to the Linux kernel and the Google Security Team mentioned that they would be participating in the evaluation of this proposal. The existing Linux kernel is currently 28mm lines of code and is one of the most complicated, mission critical software systems in the world which is why adding support for a second language is a huge deal.
Existing Linux Kernel
A kernel is a computer program that sits at the center of a computer's operating system and is the module that enables the hardware to speak to the software. It's an extremely low-level abstraction layer meaning that this software sits close to the metal.
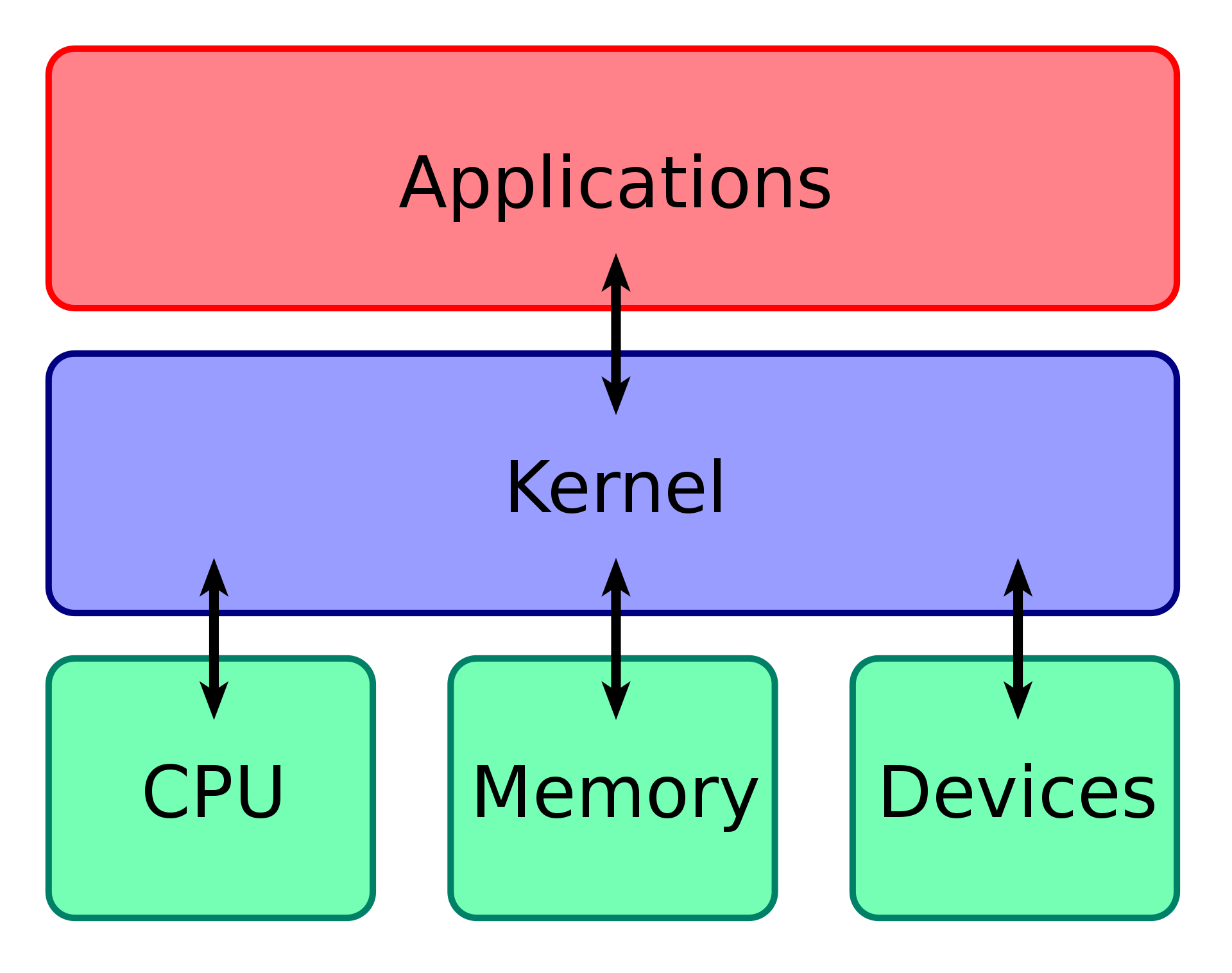
As you might expect from a piece of software that is so low-level, 95.9% of the kernel code is written in C.
Why add Rust?
C has been the lingua franca for writing kernels for almost 50 years because it offered a unique level of control and speed required by such a critical piece of software. However, because kernels have complete control of everything in the computer system and they are always resident in memory, memory safety bugs – where malicious actors can access system resources outside their allocated size and memory addresses – still regularly occur. As an example, one Microsoft engineer estimated that 70% of all vulnerabilities in Microsoft products were related to memory safety issues.
PCWalton has a good example of the difference between how memory is handled in C and how it's handled in Rust.
In C if you want to initialize a variable, x
you need to go through this two step process: first you allocate space in memory for the variable and then you initialize the value into the space that you allocated. The other issue is that part at the end free(x)
which basically tells the memory "I don't need this space in memory anymore". After running free(x)
it's not really appropriate to call x
(called at that point a dangling pointer), but C will let you use that variable.
void f() {
int *x = malloc(sizeof(int)); /* allocates space for an int on the heap */
*x = 1024; /* initialize the value */
printf("%d\n", *x); /* print it on the screen */
free(x); /* free the memory, returning it to the heap */
}
In Rust, we still need to use the pointer construct which you can see with the *x
but we don't need to free
the memory once we reach the end of the function. This "smart pointer" automagically frees allocations so you don't need to worry about forgetting to call free
and causing a memory leak.
fn f() {
let x: ~int = ~1024; // allocate space and initialize an int
// on the heap
println(fmt!("%d", *x)); // print it on the screen
} // <-- the memory that x pointed at is automatically freed here
tl;dr The semantics of Rust language will have a reduced risk of memory safety bugs and given the developer aesthetics of the language and the fact that it's been around for ~10 years and is increasingly being adopted by the largest tech companies makes it a good candidate for the kernel language extension. More broadly, this is an interesting development since projects like Solana and Ethereum utilize Rust.
h/t Anatoly Yakovenko who predicted that by 2030 50% of the Linux Kernel will be written in Rust.